python class decorator inheritance
Python 3 here just in case its important. In Python every class inherits from a built-in basic class called object.
Decorator Method Python Design Patterns Geeksforgeeks
Inheritance in Python We often come across different products that have a basic model and an advanced model with added features over and above basic model.
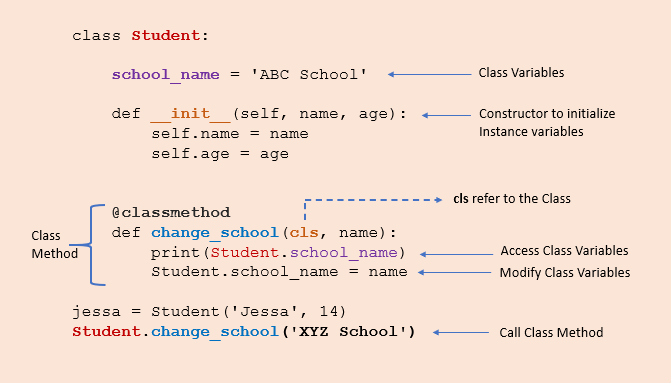
. Child class is the class that inherits from another class also called derived class. Heres my code. We can define a decorator as a class in order to do that we have to use a __call__ method of classes.
Creating Decorator inside a class in Python. These pitfalls always exist and they are also a prime reason to avoid using multiple inheritance. Python is an object oriented programming language though it doesnt support strong encapsulation.
Printbeginning funcself printend return wrapper class bazfoo. Begingroup It is probably a mistake to use an inheritance relationship between Thing and MetaThing in the first place. Thus they decorate the function with additional functionality without changing the original function.
Decorators are usually used to run code before andor after a function. The variables defined within __init__. Its a MetaThing contains Things relationship not a MetaThing is a Thing relationship.
Multiple inheritance in any language often has many pitfalls. One way of getting at the decorated class is to follow a convention that class. Klas klas for klass_method in self.
Python does some work to fix some issues with inheritance however there are numerous ways of unintentionally confusing the method resolution order mro of classes. As you might already be aware the problem arises from the fact that the name SubClassAgain in SubClassAgainprint_class is scoped to the current modules global namespaceSubClassAgain thus refers to the class _DecoratedClass rather than the class that gets decorated. This is the code Im using for testing.
When a user needs to create an object that acts as a function then function decorator needs to return an object that acts like a function so __call__ can be useful. While Python certainly can be and frequently is used as a. During Decorator creation we must take care that the function that we are defining inside the decorator must take current object reference self as a parameter and while we are accessing that decorator from child class.
Decorators allow us to wrap another function in order to extend the behaviour of the wrapped function without permanently modifying it. Python python The Star 5 returns an instance of the Star class. Return a b.
Class Inheritance allows to create classes based on other classes with the aim of reusing Python code that has already been implemented instead of having to reimplement similar code. A meta class decorator that forces creating methods from decorator-appended class def __init__ self klas. And you can use the Star class as a decorator like this.
I had the same problem here is my solution to use an inherited private decorator. Selfquux middle foo_foo__bar def quxself. Python Inheritance Inheritance allows us to define a class that inherits all the methods and properties from another class.
Im trying to properly understand how to implement inheritance when property is used and Ive already searched StackOverflow and read like 20 similar questions to no avail because the problems they are trying to solve are subtly different. Star 5 def adda b. From timeit import default_timer as timer class MetaClassDecorator object.
That instance is a callable so you can do something like. Product of 10 and 20 is 200 The sum of 10 and 20 is 30. In the following example current_user is a property method which belong to the RequestHandler class.
The standard librarys dataclass decorator is an excellent example of a sensible usage choosing decorators over inheritance. Intro to Classes in Python Class definition. However Im still a bit confused.
Create decorators by using sign. - Bruce Eckels guide to decorators - SO. You can use decorators to change classes in a way inheritance would do.
Most of the time there is another way to solve a given problem and chances are that you havent given decorators or classes let alone inheritance much thought. Parent class is the class being inherited from also called base class. After this whenever the add function is called It will always print both the product.
Introductory topics in object-oriented programming in Python and more generally include things like defining classes creating objects instance variables the basics of inheritance and maybe even some special methods like __str__. Printselfquux a baz aqux. I cant say for certain what is appropriate though since Thing is a purely hypothetical example.
In python the class statement creates the class body from the code you have written placing. They can also be used to alter the functionality of methods and. A software modelling approach of OOP enables extending the capability of an existing class to build a new class instead of building from scratch.
Python python So you can use callable classes to decorate functions. Well discuss that in a second. We can easily create decorators inside a class and it is easily accessible for its child classes.
Python-decorators-and-inheritance - SO. The first two concepts to learn about Python inheritance are the Parent class and Child class. If this is a good choice or not heavily depends on the architecture of your Python project as a whole.
The __init__ function of a class is invoked when we create an object variable or an instance of the class. Decorators are a structural design pattern that wrap the original object to add extra functionality dynamically without modifying the original object. Decorators are a very powerful and useful tool in python since it allows programmers to modify the behaviour of function or class.
Decorators classes and inheritance are fairly advanced concepts. Get-class-in-python-decorator2 - SO. Add Star 5 add Code language.
Instead of passing the add function to the class constructor we can specify decorator_class name with sign before the definition of the add function.
Understanding The Super Method In Python Askpython
Python Class Method Explained With Examples Pynative
Oop Using Inheritance To Provide One Class By Another In Python Stack Overflow
Python Property Decorator Python Property Journaldev
Python Class Method Explained With Examples Pynative
The Ultimate Class Diagram Tutorial To Help Model Your Systems Easily Class Diagram Diagram Class
How To Configure Odoo 13 On Pycharm Ubuntu 18 Technical Video 18th Make It Yourself
Python Classes And Objects Object Oriented Programming Edureka
Python Decorators Decorators In Python Scaler Topics
This Keyword In Java This Keyword In Java Is A Reference Variable That Refers To The Current Class Object In Other Words It H Java Tutorial Java Keywords
Python Exception Handling Learn Programming Keep Calm Artwork Learning
Model Constraints Python Constraints In Odoo Technical Video Python Messages
Flyweight Design Pattern Design Pattern Java Pattern Design Design
Python Class Based Decorators Intermediate Advanced Anthony Explains 195 Youtube
Abstract Factory Pattern In Java Pattern Sequence Diagram Pattern Design